Phire CMS : Open Source Content Management System
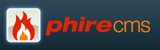
Development : Quick Reference
Here is a quick reference guide that should get you going in most use cases.
back to top ^Accessing and Rendering Pages Manually
<?php require_once('phire/bootstrap.php'); // By ID # works too // $page = new Phire_Page(8003); $page = new Phire_Page('/contact'); $page->init(); $header = new Phire_Template('Header Template'); $header->setContent('[{page_title}]', 'Page Test'); $header->setContent('[{page_sub_title}]', 'Page Test'); $header->initTemplate($page->getPageFields()); $header->render(); print($page->page_content . "\n"); $footer = new Phire_Template('Footer Template'); $footer->initTemplate(); $footer->render(); ?>back to top ^
Accessing and Using Templates Manually
<?php require_once('phire/bootstrap.php'); // By ID # works too // $header = new Phire_Template(6003); $header = new Phire_Template('Header Template'); $header->initTemplate(); $header->setContent('[{page_title}]', 'Template Test'); $header->setContent('[{page_sub_title}]', 'Template Test'); $header->render(); print(" <p>\n All of your PHP magic can happen here!\n </p>\n"); $footer = new Phire_Template('Footer Template'); $footer->initTemplate(); $footer->render(); ?>back to top ^
Accessing the Database
<?php require_once 'phire/bootstrap.php'; $db = new Phire_Db(); $db->execute("SELECT * FROM phire_pages WHERE page_id = 8002"); print($db->page_url . "<br />\n"); ?>back to top ^
A Note About the API and the Database
The API of Phire employs a pseudo active record pattern to establish a relationship to each table in the database. Upon install, there are 21 tables in the database and therefore 21 classes that represent each table in the database, like so:
Classes
|
Database Tables
|
Because each class extends the Moc10_Record component of the Moc10 PHP Library, they each inherit the same set of functionality. Here's a summary example of what is available to use to interact with the database tables via each respective class:
<?php require_once 'phire/bootstrap.php'; $feed = new Phire_Table_Feeds(); $feed->findById(9001); // - OR - $feed->findBy('feed_title', 'Google News'); // - OR - $feed->findAll(); // - OR - $feed->execute("SELECT * FROM phire_feeds WHERE feed_id = 9001"); // Then, depending on the number of row results returned, the data is accessible via object // properties like so: $feedTitle = $feed->feed_title $feedUrl = $feed->feed_url // - OR - foreach ($feed->rows as $fd) { print($fd->feed_title); } ?>back to top ^
Special Case Methods and Properties for the Table Classes
For the Phire_Table_Config class that represents the phire_config database table, the "settings" property is a publicly accessible associative array property that contains all of the configuration settings for easy recall. However, to set any new values, you’ll have to call each configuration setting separately and set it manually.
<?php require_once 'phire/bootstrap.php'; // To access a current setting $cfg = new Phire_Table_Config(); print($cfg->settings['php_version']); // To set a new value for a current setting $cfg->findById('php_version'); $cfg->value = '5.3.1'; $cfg->save(); ?>
The Phire_Table_Members class, representing the phire_members database table, has the publicly accessible method "getCount($sid)" attached to it for a quick way to get a member count for a particular site. This is especially useful as the member table grows to very large numbers.
<?php require_once 'phire/bootstrap.php'; // To get a current member count, where site_id = 2001 $members = new Phire_Table_Members(); print($members->getCount(2001)); ?>
The Phire_Table_Pages class, representing the phire_pages database table, has the publicly accessible method "getPage($id, $sid)" attached to it for a good way to get all of the data of a page, including related data in other tables, such as site and site member data, charset data, content type data, robots data, and much more. It serves as a "catch-all" data collection of all pertinent data to that page. The $id parameter can be either the page ID or the page URL and the optional $sid parameter can be the site ID of the site you which to pull the page from.
<?php require_once 'phire/bootstrap.php'; // To get a page $page = new Phire_Table_Pages('/contact'); print($page->page_requests); ?>
The Phire_Table_Sections class, representing the phire_sections database table, has the publicly accessible method "getSection($id, $sid)" attached to it for a good way to get all of the data of a section, including related data in other tables, such as site data, charset data, content type data, robots data, and much more. It serves as a "catch-all" data collection of all pertinent data to that section. The $id parameter can be either the section ID or the section URL and the optional $sid parameter can be the site ID of the site you which to pull the section from.
<?php require_once 'phire/bootstrap.php'; // To get a section $section = new Phire_Table_Sections('/blog'); print($section->section_requests); ?>
The Phire_Table_Sites class, representing the phire_sites database table, has the publicly accessible method "getSite($id)" attached to it for a good way to get all of the data of a site, including related data in other tables, such as charset data, content type data, robots data, and much more. It serves as a "catch-all" data collection of all pertinent data to that site. The optional $id parameter can be either the site ID, site domain or can be left null to automatically pull from the current site domain.
<?php require_once 'phire/bootstrap.php'; // To get a site $site = new Phire_Table_Sites(); print($site->site_domain); ?>
The Phire_Table_Templates class, representing the phire_templates database table, has the publicly accessible method "getTemplate($id)" attached to it for a good way to get all of the data of a template, including related data in other tables, such as charset data and content type data. It serves as a "catch-all" data collection of all pertinent data to that template. The $id parameter can be either the template ID or template name.
<?php require_once 'phire/bootstrap.php'; // To get a template $tmpl = new Phire_Table_Templates ('Header Template'); print($tmpl->type); ?>back to top ^
Using Sessions
Sessions are easily accessible via the Moc10_Session component of the Moc10 PHP Library (or by the old-fashioned way if you’re more comfortable with that.) Here’s an example of how to access session data using the API:
<?php require_once 'phire/bootstrap.php'; $sess = Moc10_Session::getInstance(); // For system users. print($sess->username . "<br />\n"); print($sess->user_id . "<br />\n"); print($sess->fname . "<br />\n"); print($sess->lname . "<br />\n"); print($sess->email . "<br />\n"); print($sess->last_login . "<br />\n"); print($sess->access . "<br />\n"); print($sess->open_authoring[2001] . "<br />\n"); // For site members. print($sess->username . "<br />\n"); print($sess->member_id . "<br />\n"); print($sess->fname . "<br />\n"); print($sess->lname . "<br />\n"); print($sess->email . "<br />\n"); ?>